8.1 Introduction to HTML
HTML is the backbone of every webpage. It uses tags to structure content. Below is a simple "Hello, World!" example:
Example Output:
Hello, World! This is a basic HTML snippet.
<!DOCTYPE html>
<html>
<head>
<title>Hello HTML</title>
</head>
<body>
<h1>Hello, World!</h1>
</body>
</html>
This minimal page displays a heading that reads "Hello, World!"
Additional Example:
You can change the text by modifying the content between the <h1> tags.
<h1>Welcome to My Site</h1>
8.2 Structure of an HTML Page
An HTML page begins with a doctype declaration, followed by the <html>, <head>, and <body> tags. This defines the document structure.
Example Output:
This is the body content of the page.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document Structure</title>
</head>
<body>
<p>This is the body content.</p>
</body>
</html>
8.3 Formatting Text
Format text using tags such as <b> (bold), <i> (italic), and <u> (underline). Try these examples:
Example Output:
This is bold, this is italic, and this is underlined.
<p>This is <b>bold</b>, this is <i>italic</i>, and this is <u>underlined</u>.</p>
8.4 Links
Links are created with the <a> tag. The href
attribute sets the target URL. Here's an example:
Example Output:
<a href="https://www.example.com" target="_blank">Visit Example.com</a>
target="_blank"
to open links in a new tab.
8.5 Images
Embed images using the <img> tag. Always include an alt
attribute for accessibility. Example:
Example Output:
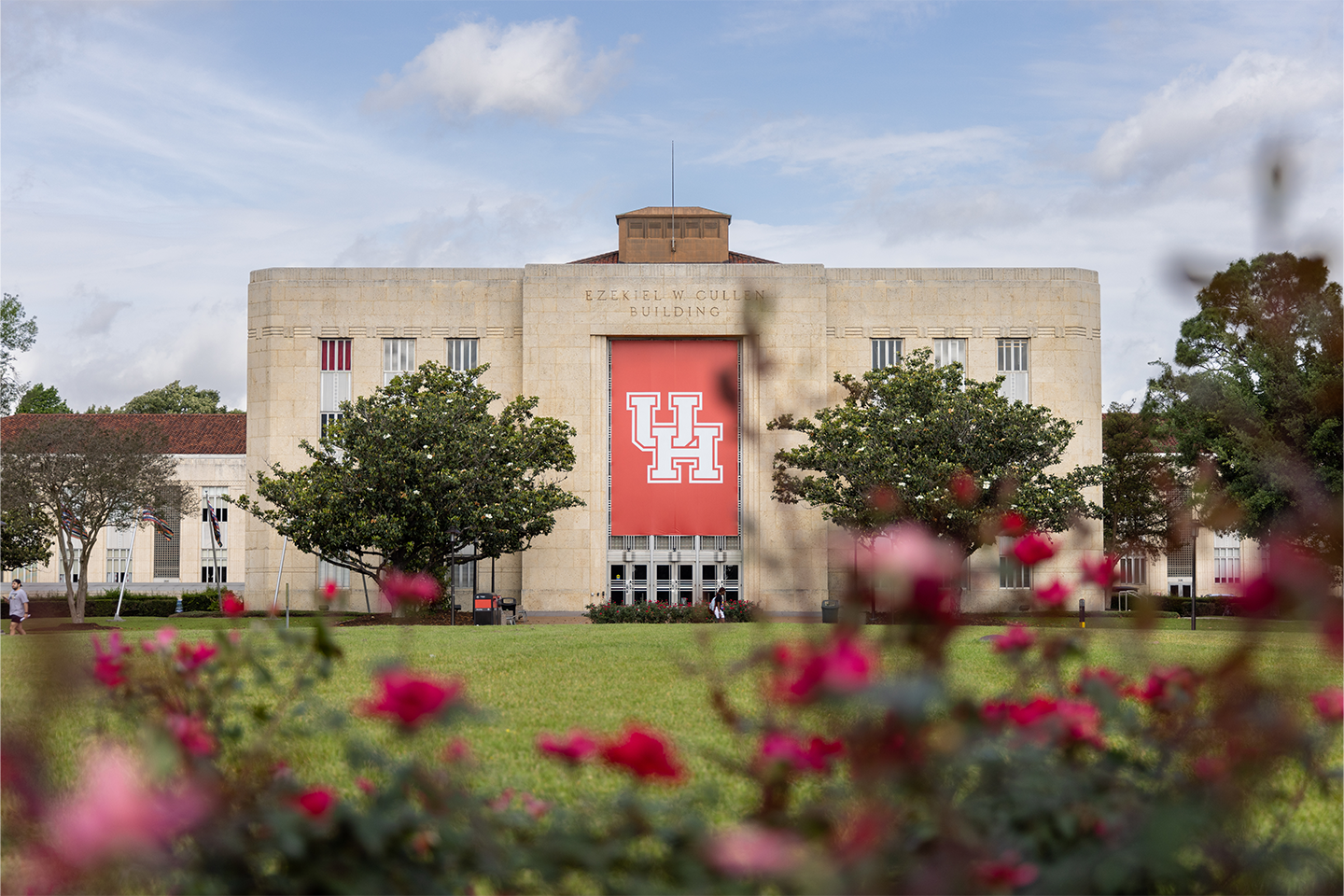
<img src="https://www.uh.edu/news-events/stories/2023/ecullen_spiff_2.png" alt="UH" />
8.6 HTML Lists
HTML supports unordered (<ul>), ordered (<ol>), and definition (<dl>) lists. Here’s an unordered list example:
Example Output (Unordered List):
- Apple
- Banana
- Cherry
<ul>
<li>Apple</li>
<li>Banana</li>
<li>Cherry</li>
</ul>
Now, try creating an ordered list:
<ol>
<li>First</li>
<li>Second</li>
<li>Third</li>
</ol>
8.7 HTML Tables
Tables display data in rows and columns. Below is an example of a table with a sortable "Score" column:
Example Output (click "Sort by Score"):
Name | Score |
---|---|
Alice | 90 |
Bob | 85 |
Charlie | 92 |
<table>
<thead>
<tr>
<th>Name</th>
<th>Score</th>
</tr>
</thead>
<tbody>
<tr><td>Alice</td><td>90</td></tr>
<tr><td>Bob</td><td>85</td></tr>
<tr><td>Charlie</td><td>92</td></tr>
</tbody>
</table>
8.8 HTML Styling
Although inline styling is possible, using CSS is recommended. Here’s an example of inline styling:
Example Output:
Inline-styled paragraph.
<p style="color: green; border: 1px solid green; padding: 5px;">
Inline-styled paragraph.
</p>
8.10 Introduction to CSS
CSS (Cascading Style Sheets) describes how HTML elements are displayed. It can be applied inline, internally, or externally. For example:
p {
color: green;
font-size: 16px;
}
This rule sets all paragraph text to green with a 16px font size.
8.11 CSS Select by Tag
Tag selectors apply styles to all elements of a given type. For example, this rule makes all <h1> elements blue:
h1 {
color: blue;
}
Now every <h1> element will have blue text.
8.12 CSS Select by Class
Class selectors, prefixed with a dot (.), allow you to apply the same style to multiple elements. This makes your CSS modular and easy to maintain.
For example, consider the following HTML:
<p class="highlight">This paragraph is highlighted.</p>
<p class="highlight">This one is also highlighted.</p>
<div class="highlight">Even divs can share the same class.</div>
And the corresponding CSS:
.highlight {
background-color: yellow;
padding: 10px;
font-weight: bold;
}
You can also combine classes on a single element:
<p class="highlight large-text">Multiple classes combined!</p>
.highlight {
background-color: yellow;
}
.large-text {
font-size: 1.5em;
}
Interactive Class Selector Example
Click the button below to show/hide a code example that demonstrates how a single class styles multiple elements. You can edit and run the code to see the live effect.
8.13 CSS Select by ID
ID selectors, prefixed with #, target a unique element. For example:
Example Output:
This text is styled using the ID "demo-id".
#demo-id {
background-color: yellow;
padding: 10px;
}
8.14 Viewing Websites
Open your HTML files locally in a browser or host them online (e.g., GitHub Pages, Netlify). Always test your site on multiple devices for compatibility.
<meta name="viewport">
tag for mobile responsiveness.
8.15 The Script Tag
The <script> tag is used to embed or link JavaScript. For example, this inline script logs a message to the console:
<script>
console.log("Hello from JavaScript!");
</script>
Background Color Picker
Use the color picker below to choose a custom background color. Click "Apply Color" to set the page's background to your selected color.
document.getElementById("apply-color").addEventListener("click", function() {
const chosen = document.getElementById("color-picker").value;
document.body.style.backgroundColor = chosen;
});
Live Code Editor Demo
Type any HTML snippet in the box below and click "Run Code" to see it rendered live:
Extended Information & Resources
Below are additional examples and syntax pointers to reinforce your understanding:
Multiple Event Listeners
This example demonstrates how to add multiple event listeners to a single element.
document.getElementById("myButton").addEventListener("click", function() {
alert("Clicked!");
});
document.getElementById("myButton").addEventListener("mouseover", function() {
console.log("Mouse over button!");
});
Simple Form Example
<form>
<label for="name">Name:</label>
<input type="text" id="name" name="name" placeholder="Your name" /><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" placeholder="you@example.com" /><br><br>
<input type="submit" class="btn" value="Submit" />
</form>